What is a Hash Map Data Structure | JavaScript
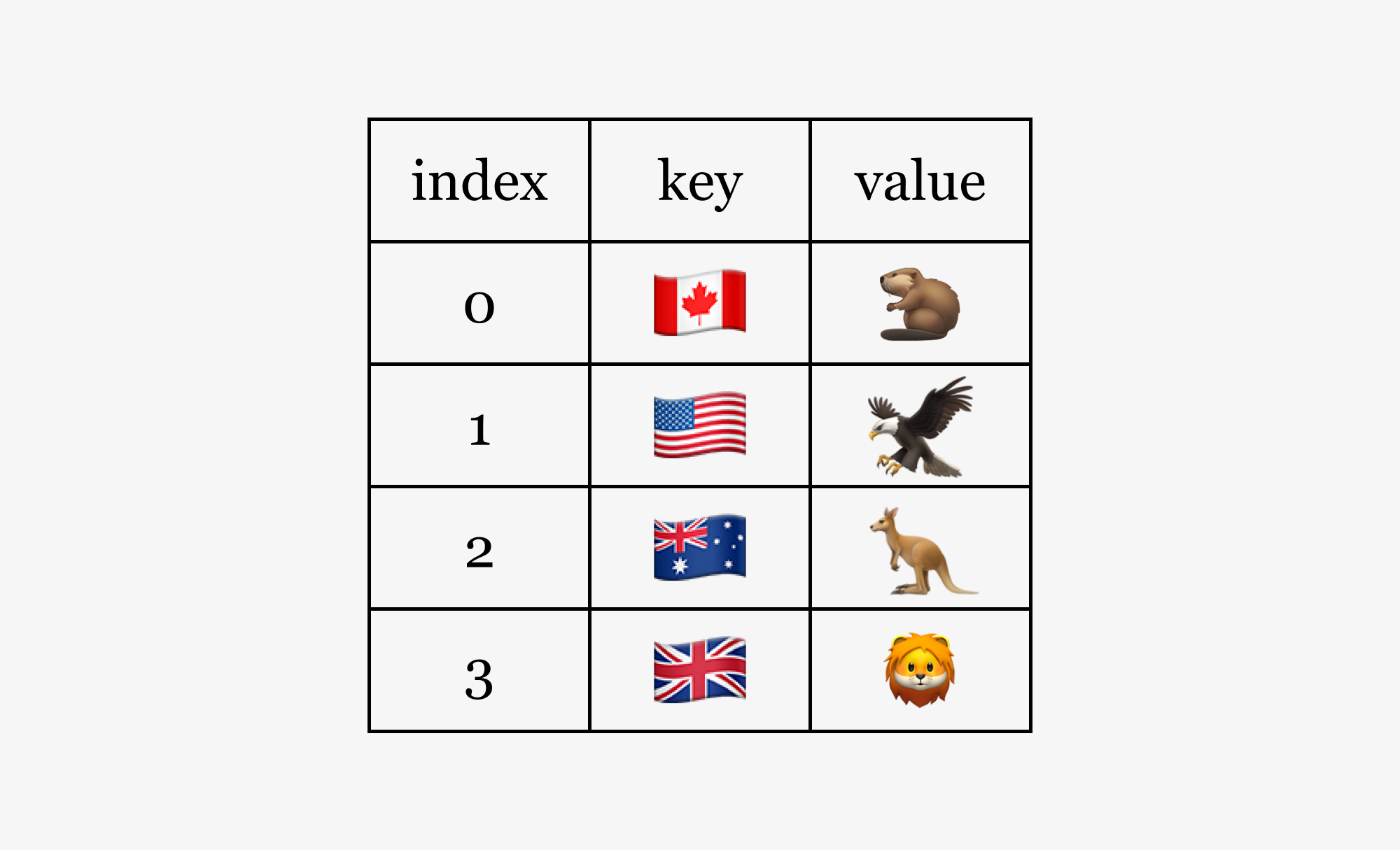
Hash Map is a non-linear data structure in computer science. Hash Maps act like key-value stores and are very efficient at saving and retrieving data.
Hash Maps
- hash function - converts a
key
into anindex
within the hash map's underlying array - hash collision - happens when a hash function returns the same
index
for several different keys - assign - saves data to a hash map at a specified by hash function
index
- retrieve - looks up the
value
in a hash map based on itskey
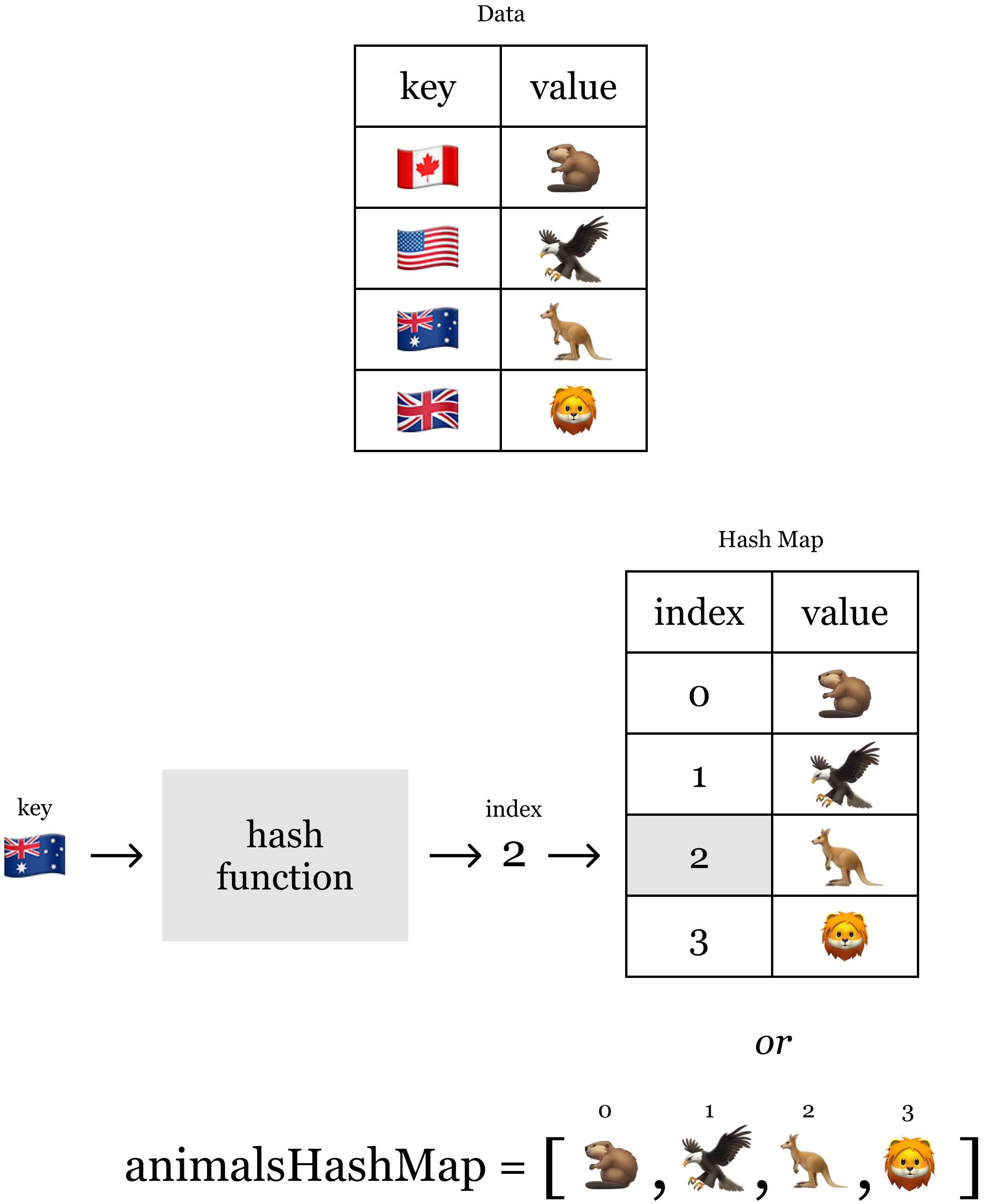
Data Types & Structures
Hash Maps are generally implemented using Arrays or Arrays of Linked Lists.
Hash Map implementation using an Array of Linked Lists
class HashMap {
constructor(size = 0) {
this.hashmap = new Array(size)
.fill(null)
.map(() => new LinkedList());
}
hash(key) {}
assign(key, value) {}
retrieve(key) {}
}