HackerRank Sales by Match | JS Solution
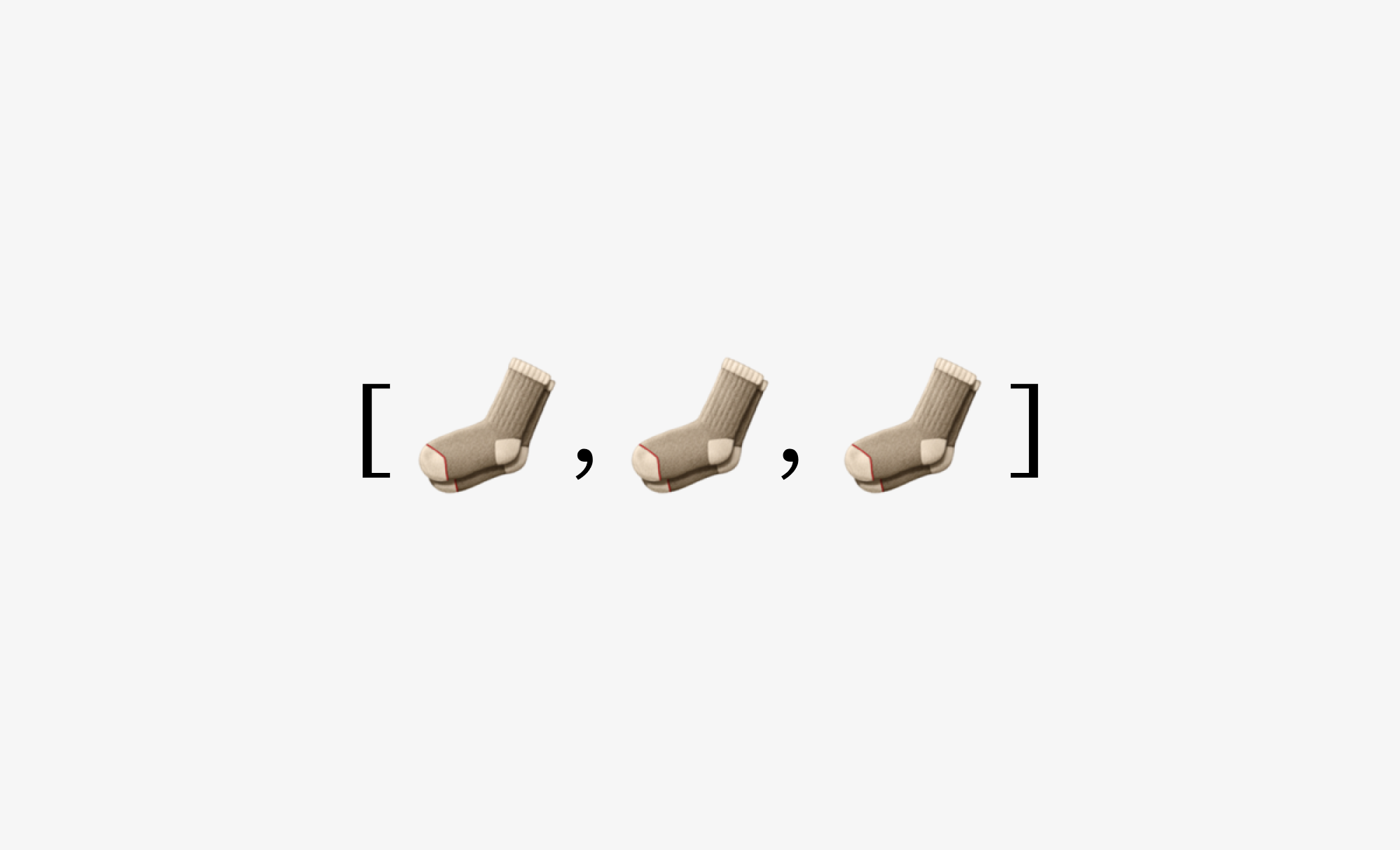
Problem
HackerRank detailed problem description can be found here.
Inputs & Outputs
/*
param {number} n
param {array of numbers} ar
returns {number} the total number of pairs
*/
Test Case
// Example used by HackerRank
n = 9;
ar = [10, 20, 20, 10, 10, 30, 50, 10, 20]
// Visual example
n = 9;
ar = ['blue', 'green', 'green', 'blue', 'blue', 'red', 'purple', 'blue', 'green']
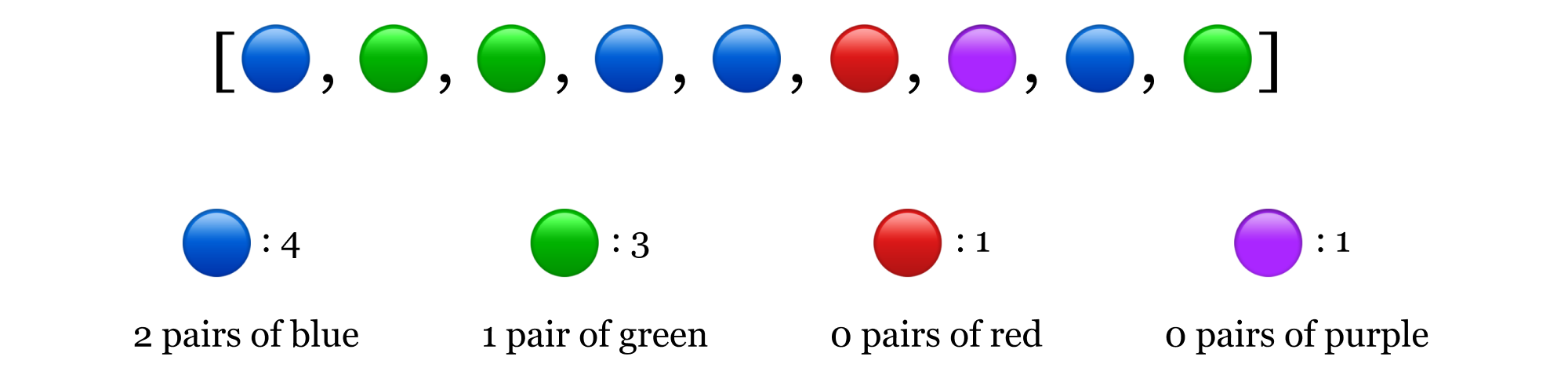
Pseudo Code
- Let's first, validate the
ar
array - Then, loop over
ar
and save each number (color) in acounterObj
object along with its quantity of occurrences, like so{ '10': 1, '20': 1 }
or{ 'blue': 1, 'green': 1 }
- If we see that there are 2 occurrences of a certain number (color) in
counterObj
, we can incrementpairs
counter and reset that number (color) value insidecounterObj
to0
in order to start counting this number (color) again from scratch - Finally, we return
pairs
JavaScript Solution
function sockMerchant(n, ar) {
// Array validation
if (!ar || ar.length !== n) {
return 0;
}
// Counting pairs
let pairs = 0;
let counterObj = {};
for (let sock of ar) {
if (!counterObj[sock]) {
counterObj[sock] = 1;
} else {
counterObj[sock] = counterObj[sock] + 1;
if (counterObj[sock] === 2) {
pairs++;
counterObj[sock] = 0;
}
}
}
return pairs;
}
Here is how our counterObj
and counterObj[sock]
would look like at the beginning of each for/of
loop iteration:
{}
undefined
{ blue: 1 }
undefined
{ blue: 1, green: 1 }
1
{ blue: 1, green: 0 }
1
{ blue: 0, green: 0 }
0
{ blue: 1, green: 0 }
undefined
{ blue: 1, green: 0, red: 1 }
undefined
{ blue: 1, green: 0, red: 1, purple: 1 }
1
{ blue: 0, green: 0, red: 1, purple: 1 }
0
3
Resources:
- Sales by Match algorithm by HackerRank
- JavaScript For Of Loop by MDN Web Docs
- Logical OR (||) by MDN Web Docs
- Working with objects by MDN Web Docs