HackerRank Let's Review | JS Solution
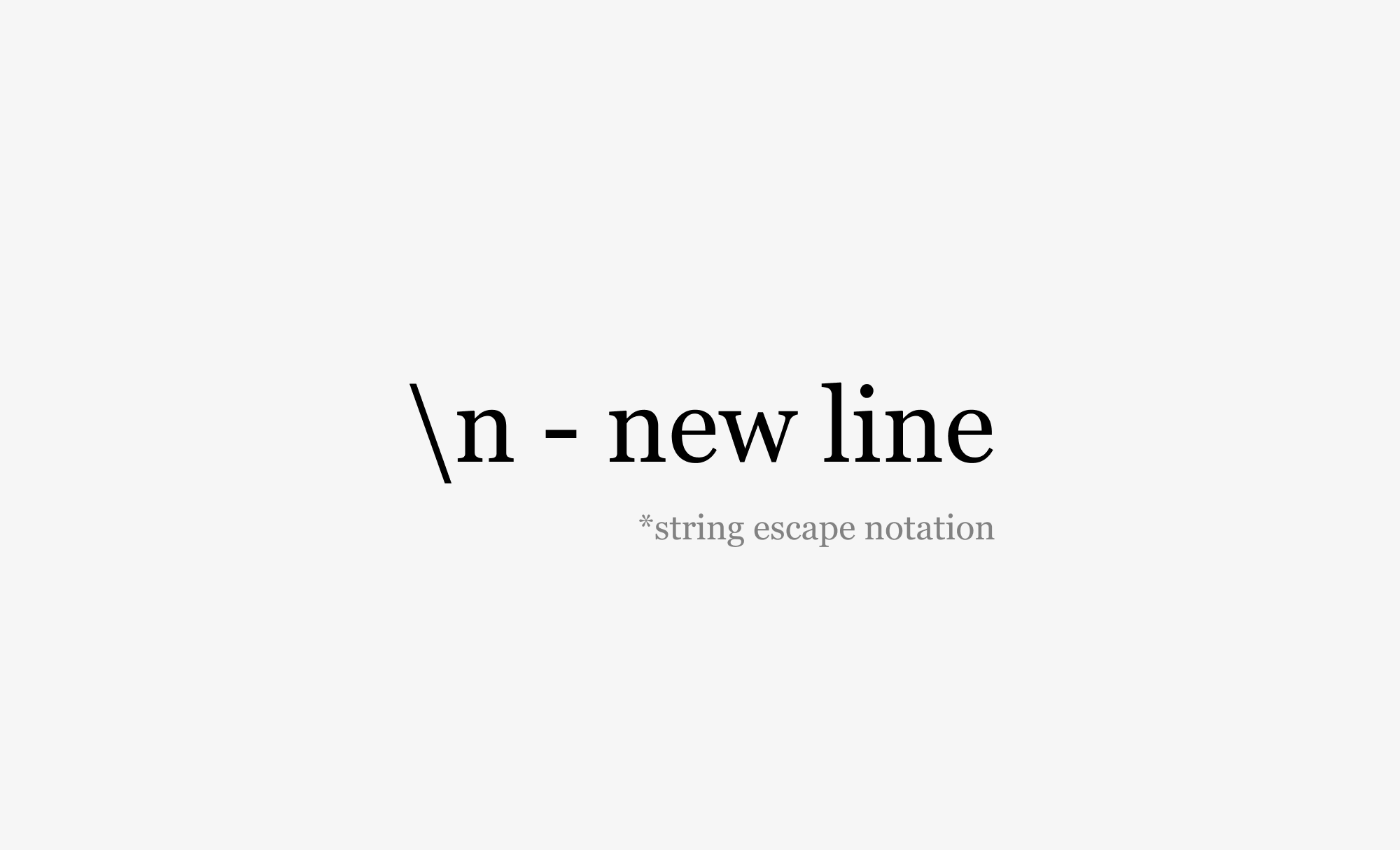
Problem
HackerRank detailed problem description can be found here.
Inputs & Outputs
/*
input {string} input
output {string} 'even' 'odd'
*/
Test Case
input = '2\nHacker\nRank';
JavaScript Solution
A bit of a 'gotcha' here is the fact that the string
we receive as an input
comes in with several new line breaks \n
and we need to make sure we get the word or character strings first before moving onto the main algorithm logic:
function processData(input) { // input = '2\nHacker\nRank'
if (!input || typeof input !== 'string') {
return;
}
let wordsArr = input.split('\n').slice(1); // wordsArr = ['Hacker', 'Rank']
wordsArr.forEach(word => {
let even = '';
let odd = '';
for (let i = 0; i < word.length; i++) {
if (i % 2 === 0) {
even = even + word[i];
} else {
odd = odd + word[i];
}
}
console.log(`${even} ${odd}`);
})
};
Resources
- Let's Review algorithm by HackerRank
- String by MDN Web Docs
- JavaScript String split() Method by W3Schools
- Array.prototype.slice() by MDN Web Docs