HackerRank Class vs Instance | JS Solution
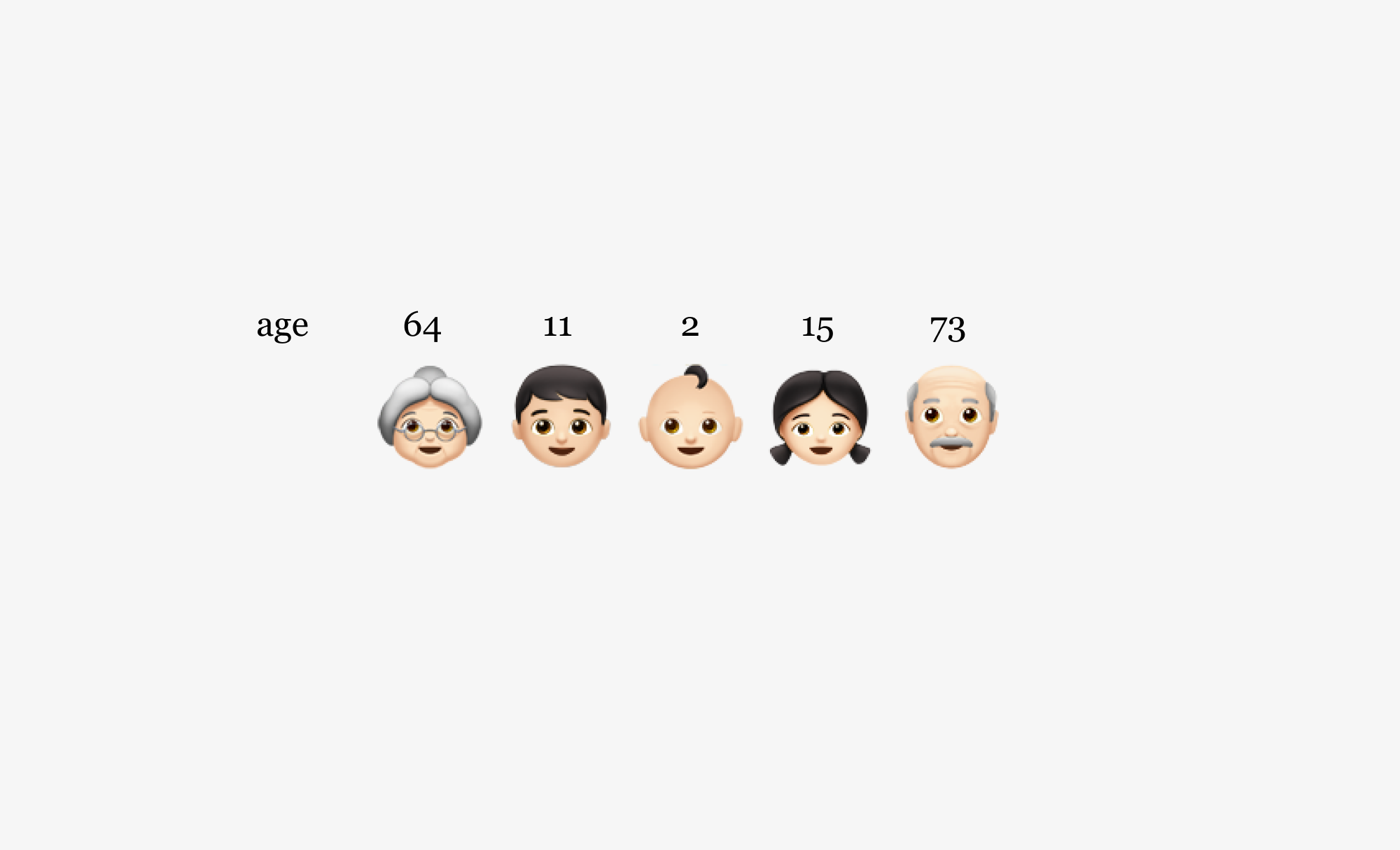
Problem
HackerRank detailed problem description can be found here.
Inputs & Outputs
/*
input {number} age
output {string}
*/
Test Case
// Different ages: 4, -1, 10, 16, 18
JavaScript Solution
function Person(initialAge) {
// Add some more code to run some checks on initialAge
let age = 0;
if (initialAge >= 0) {
age = initialAge;
} else {
console.log('Age is not valid, setting age to 0.');
}
this.amIOld = function () {
// Do some computations in here and print out the correct statement to the console
if (age < 13) {
console.log('You are young.')
} else if (age >= 13 && age < 18) {
console.log('You are a teenager.');
} else {
console.log('You are old.');
}
};
this.yearPasses = function () {
// Increment the age of the person in here
age++;
};
}
Resources
- Class vs. Instance algorithm by HackerRank
- Classes by MDN Web Docs
- JavaScript Classes by W3Schools
- The Secret Life of Objects by Marijn Haverbeke, Eloquent JavaScript