Linked List Cycle Detection | JS Solution
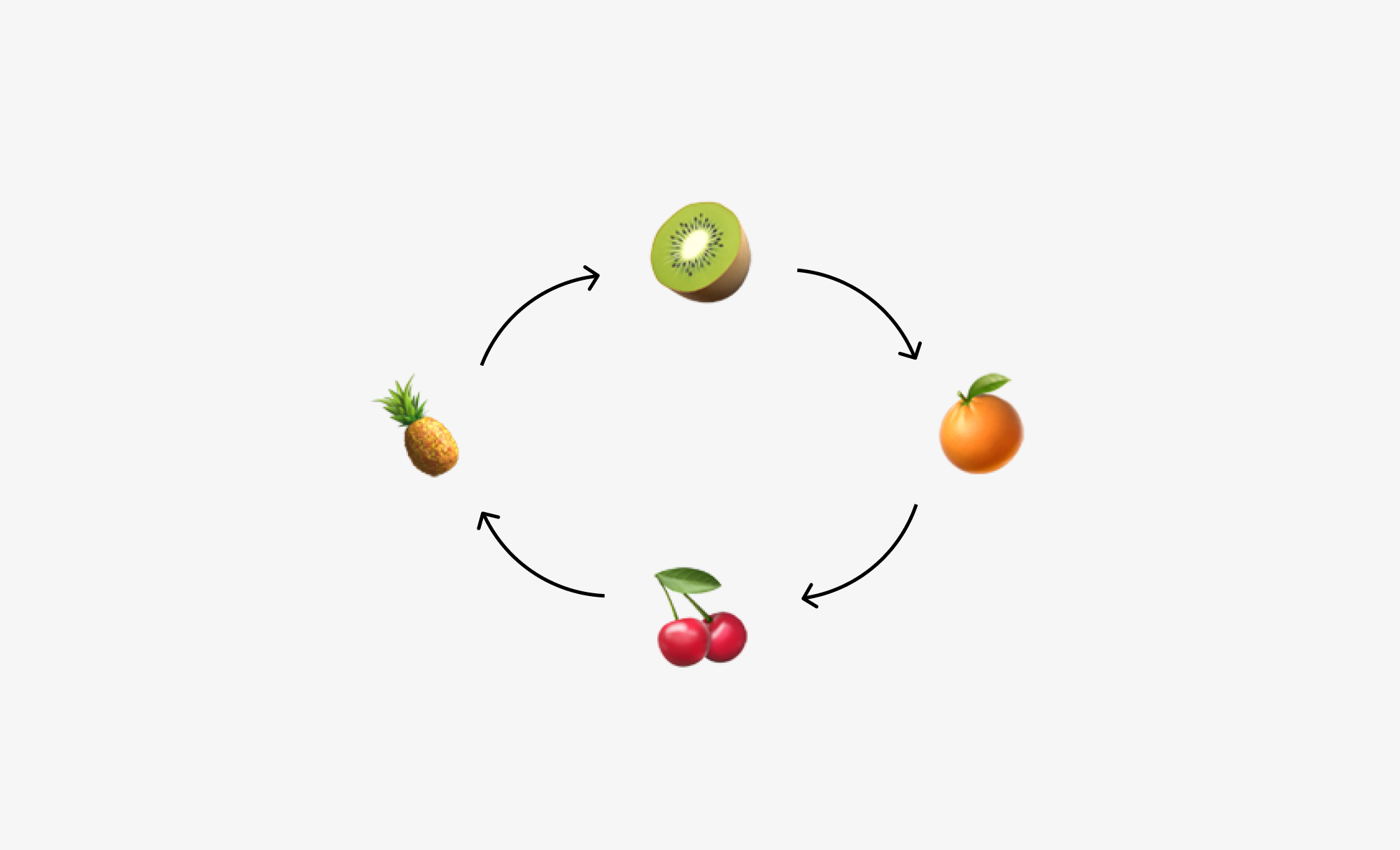
HackerRank detailed problem description can be found here.
Problem
Determine if a provided singly linked list has a cycle in it, meaning if any node is visited more than once while traversing the list.
Inputs & Outputs
/*
param {Node} head
return {number} 1 or 0
*/
Code
function hasCycle(head) {
if (!head) {
return 0;
}
let fast = head;
let slow = head;
while (fast) {
fast = fast.next;
if (fast) {
fast = fast.next;
slow = slow.next;
if (fast === slow) {
return 1;
}
}
}
return 0;
}
Sources & Resources:
- Cycle Detection Algorithm by HackerRank
- Data Structures: Linked Lists by HackerRank